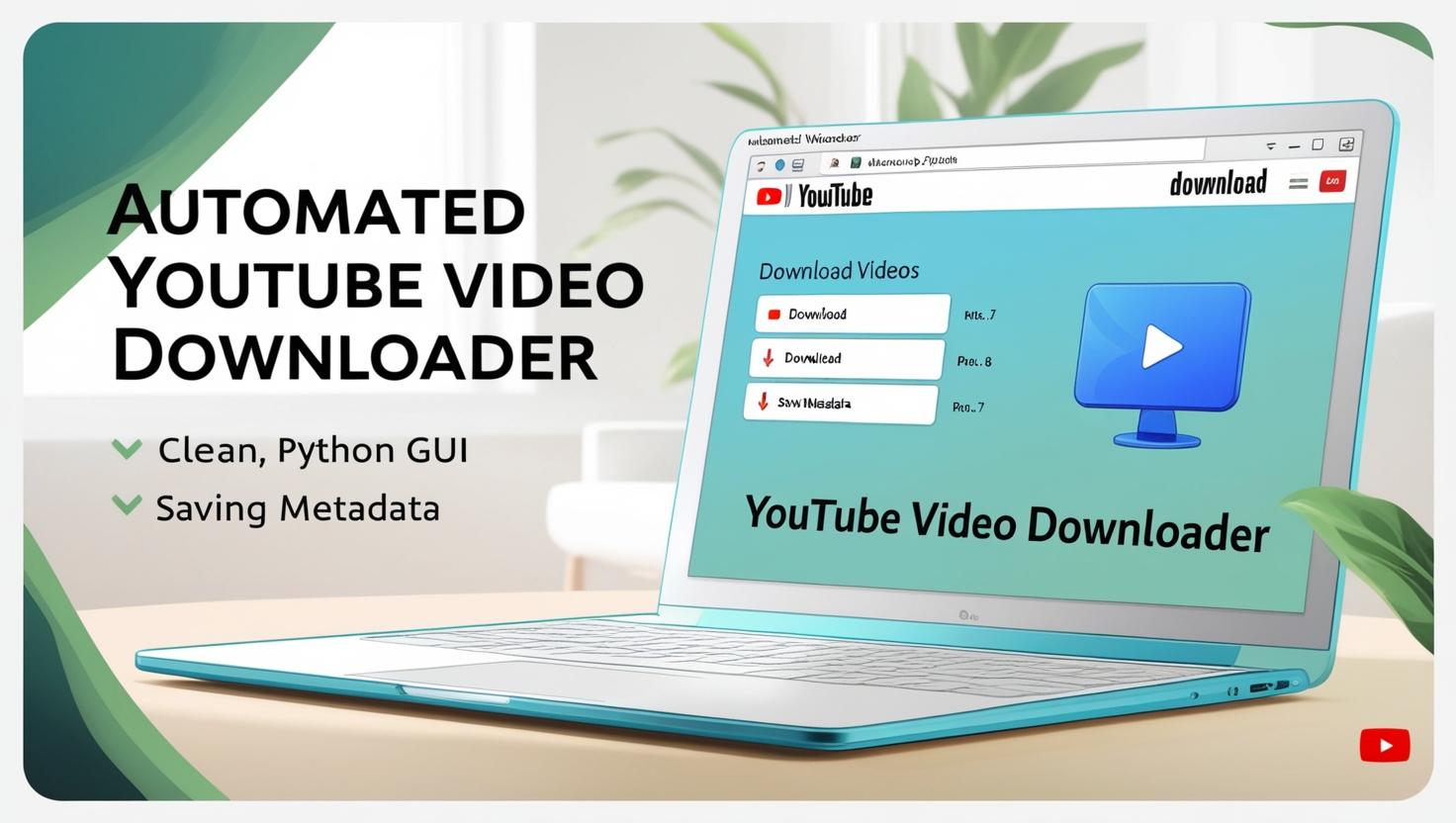
Table of Contents
Introduction
In an age where YouTube is the go-to platform for video content, there are times when you may need to download videos for offline access or for personal projects. While various online tools can help, why not create your own custom solution usingPython? In this blog post, we’ll teach you how to create an Automated YouTube Video Downloader using Python and Tkinter—a user-friendly graphical user interface (GUI) library. This downloader will not only download Automated YouTube videos but will also save essential metadata such as video titles, descriptions, and URLs in a CSV file.
Why Python is the Ideal Choice?
Python is a versatile programming language, perfect for automation tasks. With its easy-to-understand syntax and powerful libraries like yt-dlp and Tkinter, Python makes it simple to create your own video downloader and user interface. Whether you’re a beginner looking to dive into automation or an expert wanting to customize your own tool, Python provides the flexibility you need.
Features of the YouTube Video Downloader:
- Download YouTube videos with a simple interface.
- Save metadata such as video titles, descriptions, and URLs.
- Organize your downloads by selecting a folder for the saved files.
- Simple, easy-to-use GUI built with Python’s Tkinter library.
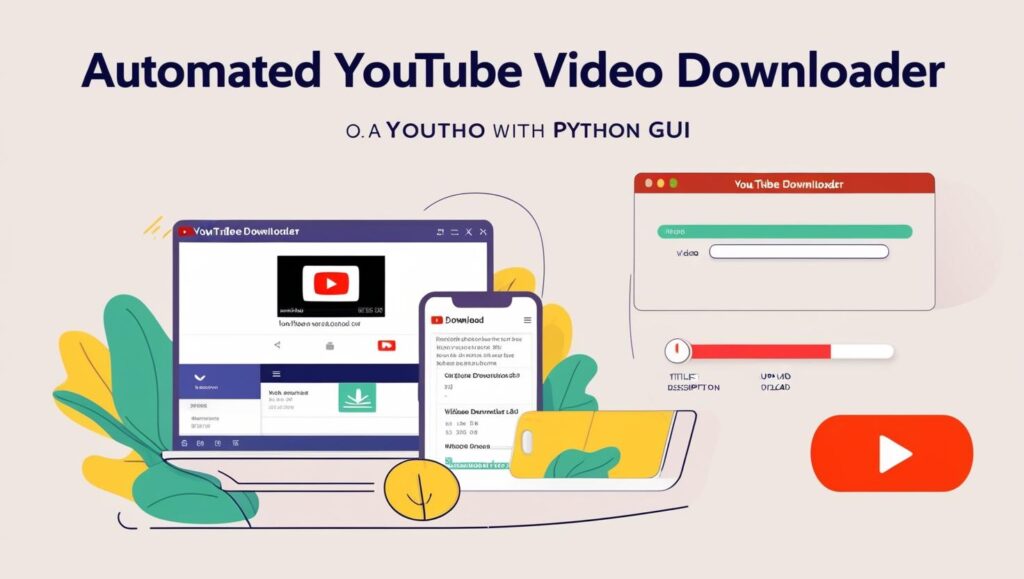
What You’ll Learn in This Guide:
By the end of this tutorial, you’ll know how to:
- Install and set up the yt-dlp library for video downloading.
- Create a GUI with Python’s Tkinter to make the tool user-friendly.
- Extract and save metadata for each downloaded video in a CSV file.
- Use this automated tool to download all the videos from a YouTube channel with a few clicks.
Step-by-Step Guide: Building Your Automated YouTube Video
Step 1: Setting Up the Environment
Before you start, make sure you have Python installed. You will also need to install two essential libraries: yt-dlp for downloading videos and Tkinter for creating the GUI. Install them using the following commands:
pip install yt-dlp
pip install tkinter
Step 2: Writing the Code
Here’s a breakdown of the Python code for this project:
import yt_dlp
import tkinter as tk
from tkinter import filedialog, messagebox
# List to store video data
video_data = []
# Function to handle video info extraction
def process_info(info_dict):
video_data.append({
'title': info_dict.get('title', ''),
'description': info_dict.get('description', ''),
'url': info_dict.get('webpage_url', '')
})
# Function to start the download process
def start_download():
channel_url = url_entry.get().strip()
if not channel_url:
messagebox.showerror("Error", "Please enter a valid YouTube channel URL.")
return
# Ask the user to select the folder to save videos and metadata
output_dir = filedialog.askdirectory(title="Select folder to save videos & CSV")
if not output_dir:
return
# Define download path and CSV file for metadata
download_path = os.path.join(output_dir, '%(title)s.%(ext)s')
csv_file = os.path.join(output_dir, 'video_metadata.csv')
# Set up yt-dlp options
ydl_opts = {
'outtmpl': download_path,
'progress_hooks': [process_info],
'format': 'best',
'merge_output_format': 'mp4',
}
try:
with yt_dlp.YoutubeDL(ydl_opts) as ydl:
ydl.download([channel_url])
# Save metadata to CSV file
if video_data:
df = pd.DataFrame(video_data)
df.to_csv(csv_file, index=False, encoding='utf-8')
messagebox.showinfo("Done", f"Downloaded {len(video_data)} videos.\nCSV saved at:\n{csv_file}")
else:
messagebox.showinfo("Info", "No new videos to download.")
except Exception as e:
messagebox.showerror("Error", str(e))
# Set up Tkinter GUI
root = tk.Tk()
root.title("YouTube Video Downloader")
tk.Label(root, text="Enter YouTube Channel URL:").pack(padx=10, pady=(10, 0))
url_entry = tk.Entry(root, width=60)
url_entry.pack(padx=10, pady=5)
download_button = tk.Button(root, text="Download Videos & Save Metadata", command=start_download)
download_button.pack(pady=10)
root.mainloop()
Step 3: Running the Application
Once the code is ready, simply run the script. Automated YouTube Video It will ask you to enter a YouTube channel URL. Once provided, it will begin downloading the videos and saving the metadata in a CSV file.
Why Build a YouTube Video Downloader with Python?
- Flexibility: Build a downloader tailored to your needs. Whether you want to download videos from specific channels or save additional metadata, you can modify the script.
- Learning: This project teaches you both Python programming and automation, skills that are highly valuable for any developer.
- Efficiency: It saves you time by automating the process of downloading videos and storing metadata.
Contact Information
If you have any questions or need further assistance with the Python Automated YouTube Video Downloaded, feel free to contact us:
Phone: +92342-1683389
Email: contact@yoursinisght.com
Download the Code
Ready to get started? Download the code and start building your own YouTube video downloader today!
Conclusion
Building your own YouTube video downloader with Python not only helps you automate video downloading, but also improves your coding skills. This project covers the basics of Python, Tkinter, and yt-dlp, making it a great resource for anyone looking to learn automation and video processing. With just a few lines of code, you can now manage your YouTube video downloads efficiently and even save important video metadata in an organized manner.
Python #yt-dlp #YouTubeDownloader #PythonGUI #Tkinter #PythonAutomation #TechTutorial”